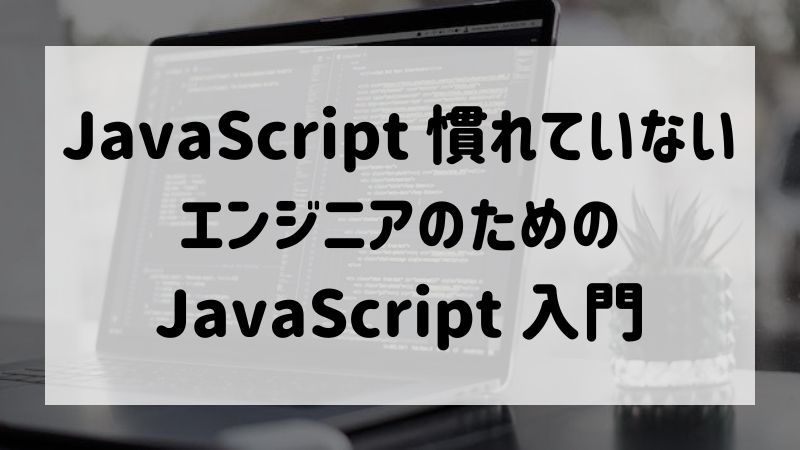
JavaScript 慣れていないエンジニアのための JavaScript 入門
目次
概要
フロント系以外のエンジニアだと、ついつい javascript の使い方を忘れてしまうことがある。私もたまに javascript から離れるとどんなメソッドがあるんだっけ?とついつい忘れてしまうことがある。 そんな悩みを解決するために本記事ではよく使う js のメソッド等を記載した。ほぼ、自分のための備忘録ではあるが・・・ 下記は node で実行。
誰向け?
- javascript そんなに使わない。けど、時には必要かも・・・・
- 多言語をよく使う人 (そのため、詳細な説明は大幅に割愛)
variable
const x = 10 // Not Redeclarable
let y = 20 //Redeclarable, Used in blocked socpe (if etc.)
var z = 30 //Redeclarable, Can be used out of blocked scope.
operator
以下、代表的な operator
=== //type and value
== //value
% //mod
!== // not type and value
!= // not value
&& // AND
|| //OR
>=
>
<=
<
... // spreads
function
modern way 1
> function sample() {
... return "x"
... }
> sample()
"x"
modern way 2
> const sample = function() {
... return "x"
... }
> sample()
"x"
arrow
> sample = () => "x"
> sample()
"x"
補足:以下、arrow を返す arrow
> res=() => (x) => x*2
[Function: res]
> double=res()
[Function (anonymous)]
> double(3)
6
分岐処理
if/else
> if (x === 1) {
... console.log("is one")
... } else if (x > 1) {
... console.log("over one")
... } else {
... console.log("under one")
... }
ternary
> const x = 3
> const y = x > 2 ? "over two": "eq and under two"
> y
"over two"
AND/OR
> const x = 0
> const y = 4
> const z = 8
> x && y
0
> x || y
8
> y && z
8
> y || z
4
AND: 左から見て、条件を満たしたら右へ、満たさなかったらその場の値を返す
OR: 左から見て、条件を満たしたらその場の値を返す、満たさなかったら右へ
switch
> switch (x) {
... case 1: {
... console.log("is one")
... }
... case 2: {
... console.log("over one and is two")
... }
... default: {
... console.log("not one or two")
... }
... }
"not one or two"
注意:switch
は if ~ else ~
と違い、 case
条件満たしても, 次の case
を満たすかを確認する。
if else のように使いたい場合は以下のやり方がある
> switch (true) {
... case ((x) => (x === 1))(x): {
... console.log("is one")
... break
... }
... case ((x) => (x > 1))(x): {
... console.log("over one")
... break
... }
... default: {
... console.log("under one")
... }
... }
"under one"
補足
case
内で関数定義と関数実行をしている
上記の 注意 でも記載したように, case
を満たしても次の case
が処理されるため、その流れを止めるために break
を記述した
array 操作
push
> var res=[];res.push(1)
1
pop
> res.pop()
1
splice
> var arr=[1,2,3,4,5,6];res = arr.splice(0,3);
[ 1, 2, 3 ]
> arr
[ 4, 5, 6 ]
slice
> arr=[1,2,3,4,5,6];
[ 1, 2, 3, 4, 5, 6 ]
> arr.slice(0,3)
[ 1, 2, 3 ]
> arr
[ 1, 2, 3, 4, 5, 6 ]
(splice は元を切る、slice は元から取る)
length
> arr.length
6
forEach
(これより先は、ほぼarrow 関数を入れる感じ)
> var res=[]
undefined
> arr=[1,2,3,4,5,6];
[ 1, 2, 3, 4, 5, 6 ]
> arr.forEach((z)=>{res.push(z)})
undefined
> res
[ 1, 2, 3, 4, 5, 6 ]
補足
forEach
の中の引数として関数を入れる。呼び出している配列(上記だと arr
)の各要素に対して実行したい処理を入力する。上記の場合だと (z) => {res.push(z)}
という関数を入力した。 z
は要素。
map
> arr.map((x)=>{return 2*x})
[ 2, 4, 6, 8, 10, 12 ]
補足
各要素を変換して新たな配列を作成したい場合のメソッド。必ず値を返してください。
filter
> arr.filter((x)=>{return x%2===0})
[ 2, 4, 6 ]
find
> arr.find(x=>x>4)
5
> arr.find(x=>x>10)
undefined
補足
最初に満たす要素を返す
findIndex
> arr.findIndex(x=>x>4)
4
> arr.findIndex(x=>x>10)
-1
補足
最初に満たす要素のインデックスを返す
reducer
> arr.reduce((stock,x)=>{return stock + x})
15
> arr.reduce((stock,x)=>{return stock + x},100)
115
補足
reduce(集計関数、初期値)
である。集計関数
は (合算値, 要素) => {return 新合算値}
と定義する。
keys (index)
index のみ返す
> var tf_arr=[true,false,true,false,true,true]
> tf_arr.map((_,i)=>i)
[ 0, 1, 2, 3, 4, 5 ]
fill (補完)
> Array(4).fill(true)
[ true, true, true, true ]
zip
各要素の結合
> zip=(x,y)=>{return x.map((v,k)=>{return [v,y[k]]})}
> zip([1,2,3],[4,5,6])
[ [ 1, 4 ], [ 2, 5 ], [ 3, 6 ] ]
enumerate
> const tf_arr = ["a","b","c"]
> tf_arr.forEach((v,k) => {console.log(k,v)})
0 "a"
1 "b"
2 "c"
spread
> const arr_x = [1, 2, 3]
> const new_x = 4
> [...arr_x, new_x]
[1, 2, 3, 4]
Object 操作
keys
key のみ取得
> dict={"a":1,"b":2}
> Object.keys(dict)
[ 'a', 'b' ]
values
value のみ取得
> Object.values(dict)
[ 1, 2 ]
entries
key value 取得
> Object.entries(dict)
[ [ 'a', 1 ], [ 'b', 2 ] ]
応用:entries と forEach の組み合わせ
各 key, value で操作したい場合は以下の通り
> Object.entries(dict).forEach(([k,v]) => {console.log(k,v)})
a 1
b 2
for loops
iterrate
> for (const x in tf_arr){console.log(x)}
0
1
2
3
4
5
まとめ
以上がよく使う js のメソッドでした。他にも if, case, async 色々とあるが、上記の知識があればあとはググるなり、聞くなりすることで解決するのではと思います。